Integrating with SDK
The Sensible Weather Javascript SDK is designed to allow you to seamlessly generate Weather Guarantee Quotes from within your client-side code.
Auth
The Javascript SDK is authenticated using CORS policies combined with a limited security footprint. The SDK is only permitted to generate quotes, but you will still need to accept the quote within your system's backend by using the Sensible Weather API.
To interact with the Javascript SDK, your app must be able to authorize using our auth API. A partner_id
or product_id
is provided via shared secure vault. Your CORS domains must also be whitelisted.
Installing the SDK
To begin using the SDK, copy the following into the HTML header of the desired page.
<script type='module' src='https://static.sensibleweather.io/js-sdk/v1.5.1/build/sensible-sdk.esm.js'></script>
<script nomodule src='https://static.sensibleweather.io/js-sdk/v1.5.1/build/sensible-sdk.js'></script>
<link rel="stylesheet" href="https://static.sensibleweather.io/js-sdk/v1.5.1/assets/css/fonts.css" />
Once the SDK has been installed on your page, you can access it as a global object named Sensible
.
To configure the SDK, call the Sensible.configure
method, passing in your partner_id
, product_id
, and an optional third argument to specify a connection to the sandbox environment.
<script>
// Production Configuration
document.addEventListener('DOMContentLoaded', () => {
Sensible.configure('your_partner_id', 'your_product_id');
});
</script>
<script>
// Sandbox Configuration
document.addEventListener('DOMContentLoaded', () => {
Sensible.configure('your_partner_id', 'your_product_id', 'sandbox');
});
</script>
Creating a Quote
In your checkout flow, use the Sensible SDK to create a quote.
const quote = await Sensible.quote.createQuote({
coverageStartDate: "2024-01-01",
coverageEndDate: "2024-01-05",
currency: "usd",
exposureName: "Example Exposure Name",
exposureLatitude: 37.77664018315584,
exposureLongitude: -111.63836828825272,
exposureTotalCoverageAmount: 10,
langLocale: "en-us"
});
The created quote will provide you with all of the information needed to display to your customer under the quoteData
namespace.
The quote object also provides suggested plain language to be used when incorporating the quote into your checkout UI. The main
namespace includes language suitable for a simple inline item, while the details
namespace includes language suitable for either a modal or separate page.
interface Quote {
quoteData: {
id: string;
pricePerDay: number;
totalPrice: number;
dailyCoverageStartHour: number;
dailyCoverageEndHour: number;
sortedTiers: {
hours: number;
reimbursementRate: number
}[];
exposureProtections: {
name: string;
unit: string;
upperThreshold?: number;
lowerThreshold?: number;
}[];
currency: "usd" | "eur" | "gbp";
},
main: {
title: string;
suggested_price: string;
suggested_price_total: string;
body: string;
action: string;
open_details: string;
terms_conditions: string;
privacy: string;
},
details: {
title: string;
summary_heading: string;
summary_times: string;
summary_tiers: string[];
summary_subheading: string;
summary_body: string;
summary_explainer: string;
steps_heading: string;
step_1_heading: string;
step_1_body: string;
step_2_heading: string;
step_2_body: string;
step_3_heading: string;
step_3_body: string;
summary_body: string;
suggested_price: string;
suggested_total_price: string;
action: string;
close: string;
terms_conditions: string;
privacy: string;
}
}
Suggested Plain Language Usage
Bolded plain language keys are required. They contain content for legal compliance or reference a specific weather peril (rain in this case). Once weather peril keys are in place they can be used to dynamically support content for other weather perils such as high temperature, precipitation, etc.
Single Day Weather Guarantee (embedded component)
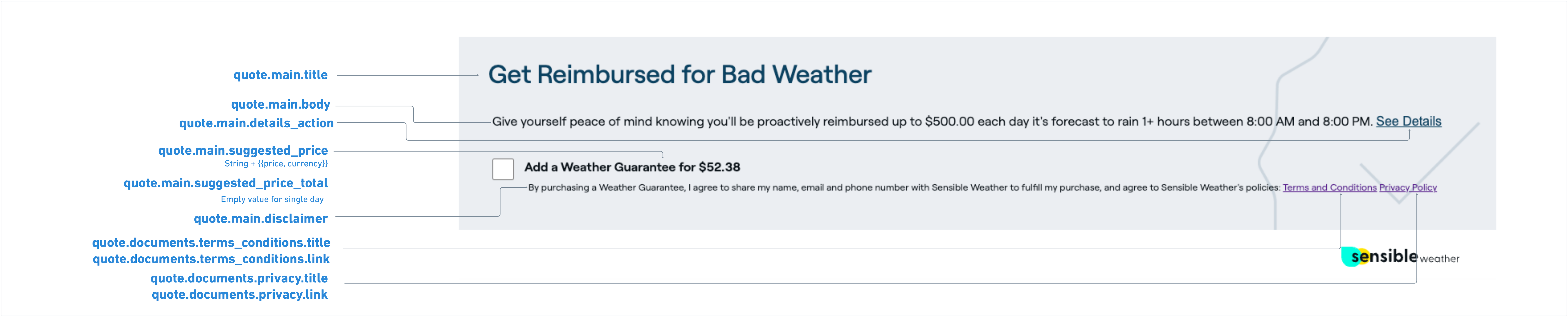
quote.main.disclaimer
should be displayed next to any “Add Weather Guarantee” CTA to make it clear that by purchasing a Weather Guarantee a customer is agreeing to our legal policies.
quote.main.suggested_price
and quote.main.suggested_price_total
are used for both single and multi day Weather Guarantees.
Single day will return an empty value for quote.main.suggested_price_total
since the price per day and total amount is the same.
Multi Day Weather Guarantee (embedded component)

Multi day will return both the per day and total price for quote.main.suggested_price
and quote.main.suggested_price_total
.
Single Day Weather Guarantee (popup module)
Customers see the popup module after clicking "See Details" in the embedded component.
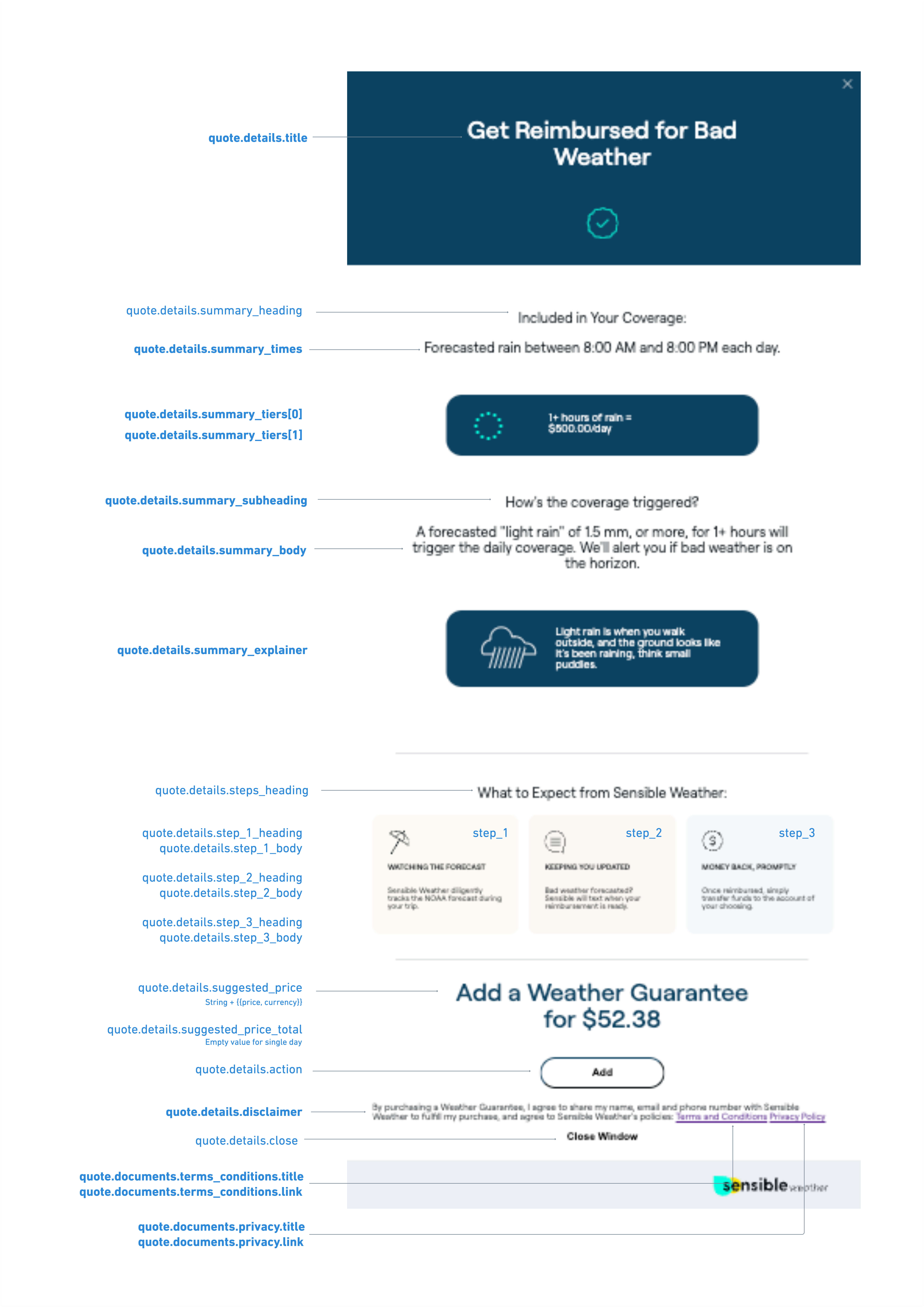
We recommend using quote.details.summary_body
to display 1.5mm. quote.details.summary_body_alt_unit
makes it possible to show other units such as m, cm, and Celsius. alt_unit
will currently display 0.15 cm.
Multi Day Weather Guarantee (popup module)
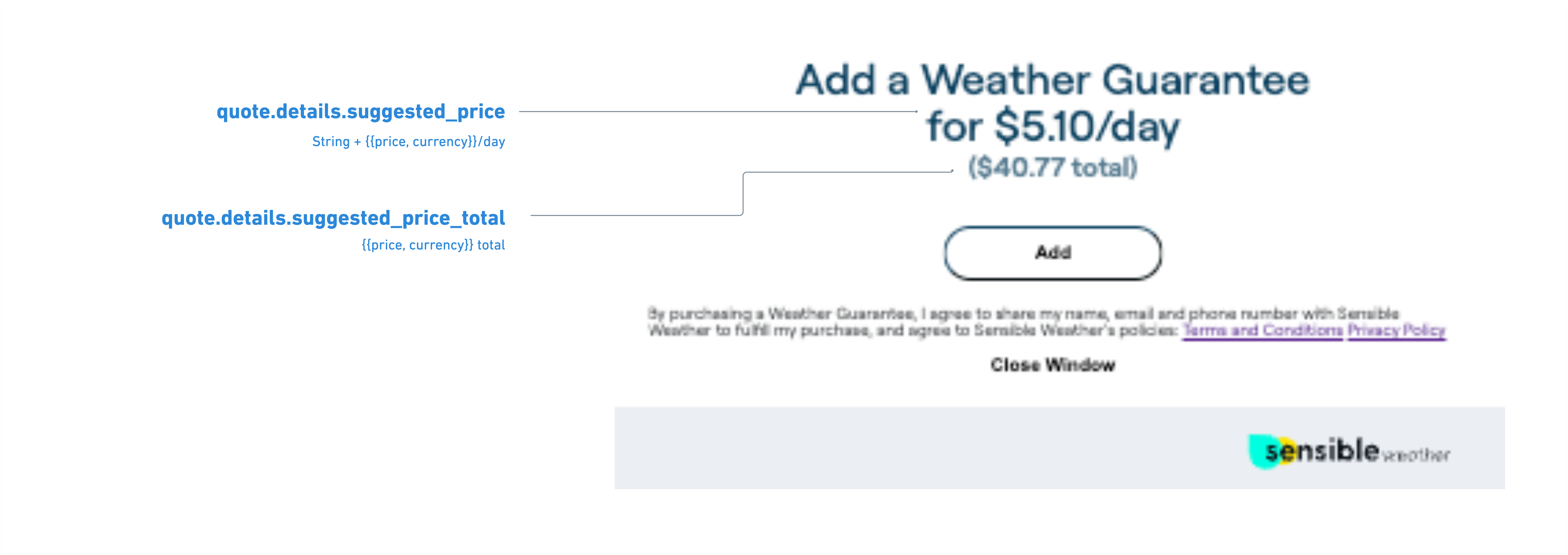
Accepting or Declining a Quote
Once your customer has selected a quote created by the Sensible Weather SDK, you will be able to complete the process of Accepting or Declining the quote using the Sensible Weather API within your backend. To do so, follow the steps in the Integrating with API guide, passing the ID value from the SDK generated quote.
Updated 11 months ago